Q1: What’s a[1:1] if a is a string of at least two characters? And what if string is shorter?
A1: In Python, when you use slicing, the start index is inclusive and the end index is exclusive. So, if `a` is a string of at least two characters, `a[1:1]` will be an empty string because it starts and ends at the same index without including the end index. If the string is shorter (i.e., has only one character), `a[1:1]` would still be an empty string, because the index 1 does not exist in the string.
Q2: What are the two ways to add something to a list? How are they different?
A2: In Python, you can add an element to a list using the `append()` or `extend()` methods, or using the `insert()` method.
The `append()` method adds an element to the end of the list. If you append a list to another list, the whole list gets appended as a single element.
The `extend()` method also adds elements to the end of a list, but if you extend a list with another list, each element of the second list gets individually appended to the first list.
The `insert()` method inserts an element at a specific position in the list.
Q3: What are the two ways to remove something from a list? How are they different?
A3: You can remove an element from a list in Python using the `remove()` or `pop()` methods.
The `remove()` method removes the first matching value from the list. If the value is not found, it throws a ValueError.
The `pop()` method removes an element at a specific position in the list and returns it. If no index is specified, it removes and returns the last element in the list. If the index is out of range, it throws an IndexError.
Q4: What is the difference between a list and a tuple?
A4: Lists and tuples in Python are quite similar in that they both can store multiple items in a single variable. The major difference is that lists are mutable, meaning that their contents can be changed after creation, while tuples are immutable, meaning that once a tuple is created, it cannot be changed.
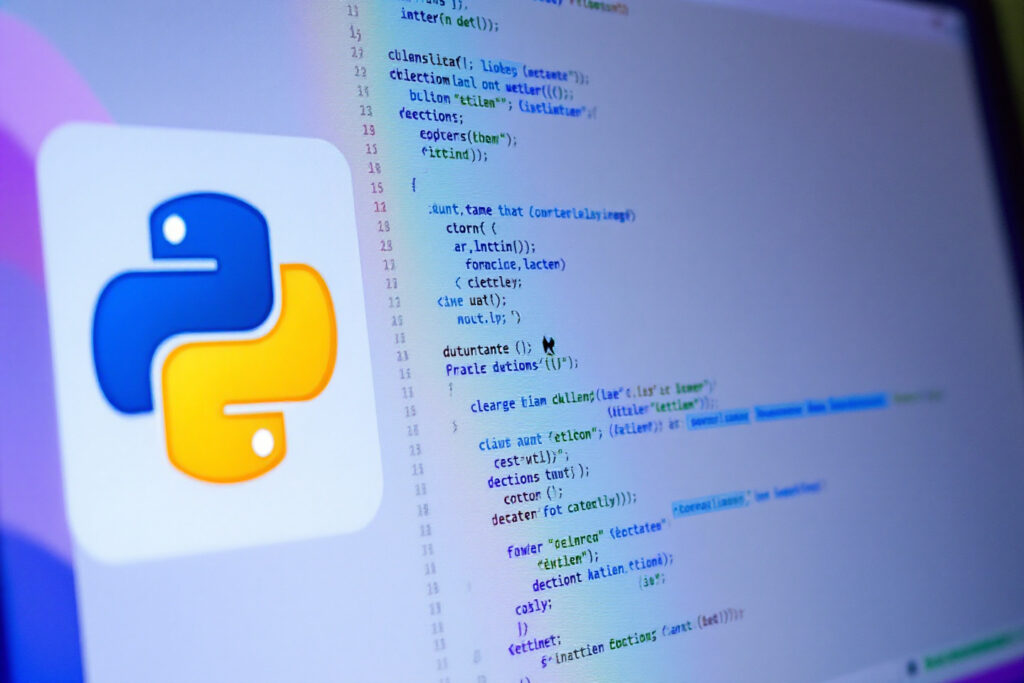